Real-time Collaboration (multiplayer text editor)
Let's see how you can add Multiplayer capabilities to your BlockNote setup, and allow real-time collaboration between users (similar to Google Docs):
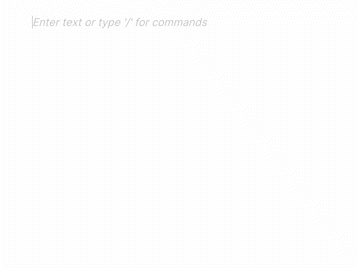
Try the live demo on the homepage
BlockNote uses Yjs for this, and you can set it up with the collaboration
option:
import * as Y from "yjs";
import { WebrtcProvider } from "y-webrtc";
// ...
const doc = new Y.Doc();
const provider = new WebrtcProvider("my-document-id", doc); // setup a yjs provider (explained below)
const editor = useBlockNote({
// ...
collaboration: {
// The Yjs Provider responsible for transporting updates:
provider,
// Where to store BlockNote data in the Y.Doc:
fragment: doc.getXmlFragment("document-store"),
// Information (name and color) for this user:
user: {
name: "My Username",
color: "#ff0000",
},
},
// ...
});
Yjs Providers
When a user edits the document, an incremental change (or "update") is captured and can be shared between users of your app. You can share these updates by setting up a Yjs Provider. In the snipped above, we use y-webrtc which shares updates over WebRTC (and BroadcastChannel), but you might be interested in different providers for production-ready use cases.
- Liveblocks A fully hosted WebSocket infrastructure and persisted data store for Yjs documents. Includes webhooks, REST API, and browser DevTools, all for Yjs
- PartyKit A serverless provider that runs on Cloudflare
- Hocuspocus open source and extensible Node.js server with pluggable storage (scales with Redis)
- y-websocket provider that you can connect to your own websocket server
- y-indexeddb for offline storage
- y-webrtc transmits updates over WebRTC
- Matrix-CRDT syncs updates over Matrix (experimental)
- Nostr-CRDT syncs updates over Nostr (experimental)
Liveblocks
Liveblocks provides a hosted back-end for Yjs which allows you to download and set up a real-time multiplayer BlockNote example with one command.
npx create-liveblocks-app@latest --example nextjs-yjs-blocknote-advanced
You can also try the same example in a live demo. To start with Liveblocks and BlockNote make sure to follow their getting started guide.
Partykit
For development purposes, you can use our Partykit server to test collaborative features. Replace the WebrtcProvider
provider in the example below with a YPartyKitProvider
:
// npm install y-partykit
import YPartyKitProvider from "y-partykit/provider";
const provider = new YPartyKitProvider(
"blocknote-dev.yousefed.partykit.dev",
// use a unique name as a "room" for your application:
"your-project-name",
doc
);
To learn how to set up your own development / production servers, check out the PartyKit docs and the BlockNote + Partykit example.